For loop behavior in PHP
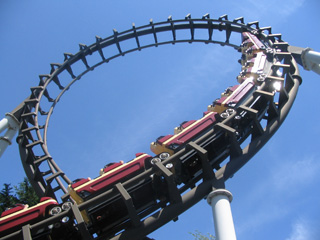
I always asked myself how often a function gets evaluated if you place it in the break condition of a for loop (or a for-each loop). Unfortunately I have always been too lazy to look for an answer – until today.
Let’s have a look at an implementation in PHP:
<?php
function times() {
echo "evaluation\n"; // report evaluation
return 5;
}
echo "# for loop:\n\n";
for ($i = 0; $i < times(); $i++) {
echo $i . "\n";
}
function set() {
echo "evaluation\n"; // report evaluation
return array(0, 1, 2, 3, 4);
}
echo "\n# for-each loop:\n\n";
foreach (set() as $i) {
echo $i . "\n";
}
This will print
# for loop:
evaluation
0
evaluation
1
evaluation
2
evaluation
3
evaluation
4
evaluation
# for-each loop:
evaluation
0
1
2
3
4
As we can see, PHP evaluates the break condition of a for loop at each iteration, whereas in a foreach loop, the set of values over which the loop iterates, only gets evaluated once.
So, if you use a function with an expensive calculation as a break condition in a for loop, just store that value in a temporary variable. E.g.: